The error Call to a member function getCollectionParentId() on null is common in PHP-based applications, particularly when working with objects and database queries. This error usually indicates that you’re trying to call a method on a variable or object that hasn’t been initialized properly. If left unresolved, it can lead to significant issues within your code.
In this guide, we will break down the error, its causes, and how to resolve it through various best practices.
What Does the Error “Call to a Member Function getCollectionParentId() on Null” Mean?
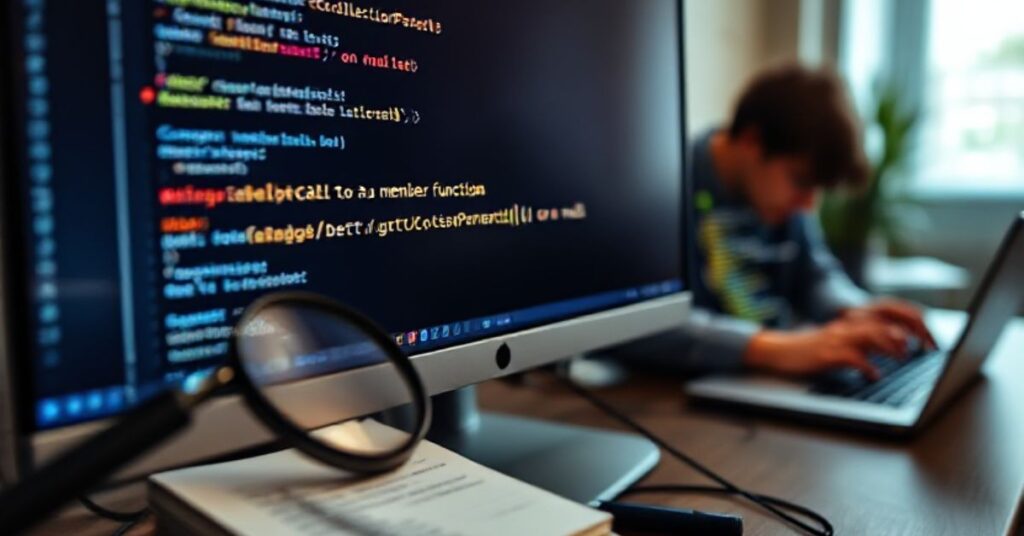
The error “Call to a member function getCollectionParentId() on null” occurs when your code attempts to call the getCollectionParentId() method on a variable that is null, meaning the variable has not been assigned an object or value that can invoke the method.
In object-oriented programming, when an object is expected to be passed to a function or method, it must be properly instantiated. If the object is not created or is assigned as null, calling a method on it will cause a runtime error.
This error is essentially telling you that the variable you are trying to work with doesn’t hold an object or reference that can handle the method call. It’s important to handle this scenario by checking whether the object is initialized (not null) before attempting to use its methods.
This could be due to database queries returning no results, improper object instantiation, or errors in the flow of data between functions or processes. Ignoring or missing this issue can cause unpredictable behavior or crashes in your application.
What Are the Common Causes of This Error?
There are several potential reasons this error can occur:
- Uninitialized Objects: If you try to call the method on an object that hasn’t been instantiated yet, PHP will throw this error.
- Null Variables: The variable you’re working with was expected to hold an object but instead is null. This is common when database queries fail to return data or when objects are not properly passed between functions or methods.
- Database Query Failures: A database query might return null when no matching records are found. If the result is not checked before calling methods on it, this error may occur.
- Incorrect Data Flow: The object or variable may not be passed correctly, overwritten, or accessed in an improper scope, leading to a null value when attempting to use it.
- Framework-Specific Issues: This error is commonly seen in various frameworks, including ConcreteCMS or Magento, especially when dealing with collections, pages, or models that fail to load correctly.
How Can I Prevent This Error in My Code?
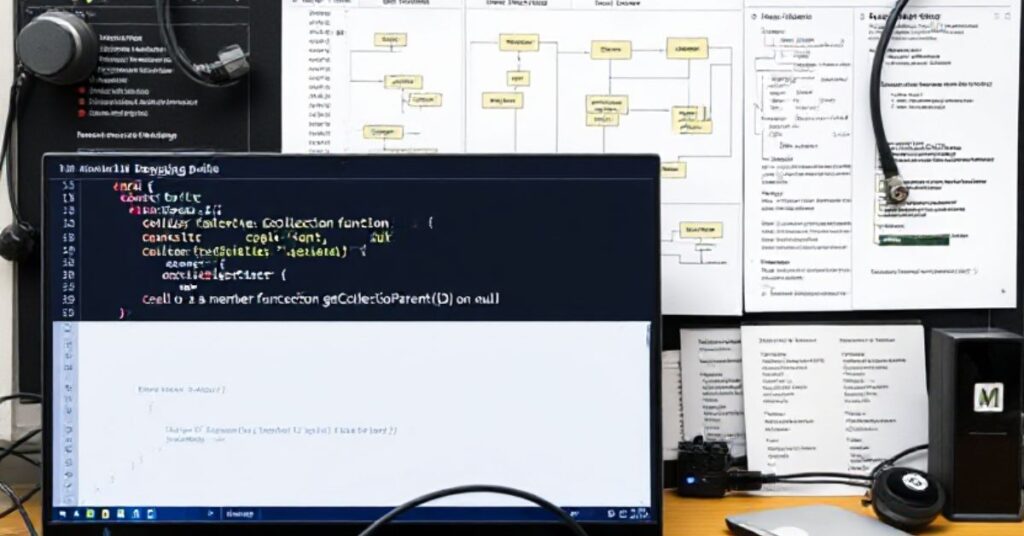
To prevent this error from occurring, follow these guidelines:
Check for Null:
Always verify that an object or variable is not null before invoking any methods on it. Using an if statement ensures that the method is only called when the object is initialized.
php
if ($collection !== null) {
$parentId = $collection->getCollectionParentId();
} else {
echo “Collection is null.”;
}
Proper Initialization:
Ensure that all objects are instantiated before use. This means making sure that your objects are created properly by checking their constructors and initialization routines.
Validate Data:
Before calling methods that rely on data from external sources (like databases or APIs), validate that the data is not empty or null. This extra check can prevent the error from occurring unexpectedly.
Error Handling:
Implement appropriate error handling to gracefully manage scenarios where an object might be null. This could involve using try-catch blocks in PHP to handle exceptions more effectively.
Can You Provide an Example of How to Handle This Error?
Certainly! Here’s a simple example that demonstrates how to check for null before calling the getCollectionParentId() method:
php
$collection = getCollectionById($collectionId); // Function that retrieves a collection
if ($collection !== null) {
$parentId = $collection->getCollectionParentId();
} else {
// Handle the case where $collection is null
echo “The requested collection does not exist.”;
}
This ensures that the method is only called when $collection is valid, avoiding the error.
What Should I Do if a Database Query Returns Null?
If you’re dealing with a situation where a database query is expected to return an object but instead returns null, here’s how you can address it:
Check Query Results:
Always check the results of a database query to ensure it has returned the expected data. This will help identify if the query is working correctly.
php
$queryResult = $db->query(“SELECT * FROM collections WHERE id = $id”);
if ($queryResult && $queryResult->num_rows > 0) {
$collection = $queryResult->fetch_object();
} else {
echo “No collection found.”;
}
Handle Empty Results:
If no results are returned, add a fallback logic to manage such cases. For instance, you could display a message or return a default object to avoid errors.
Log Errors:
Always log any issues with database queries so that you can investigate why the query did not return the expected results.
How Can I Debug This Error Effectively?
Debugging this error can be challenging, but with the right tools, you can identify and fix the issue quickly:
- Use Debugging Tools: Tools like Xdebug allow you to step through your code line by line, inspecting variables and the flow of execution to pinpoint where things go wrong.
- Add Logging: Insert logging statements to track the values of variables, especially those involved in the error. This can help identify which variable is null when the method is called.
php
error_log(‘Collection object: ‘ . var_export($collection, true));
- Check Stack Traces: When an error occurs, PHP typically provides a stack trace. This trace can help you identify where in the code the error occurred, allowing you to focus your debugging efforts on that section of the code.
Is This Error Specific to Certain PHP Frameworks?
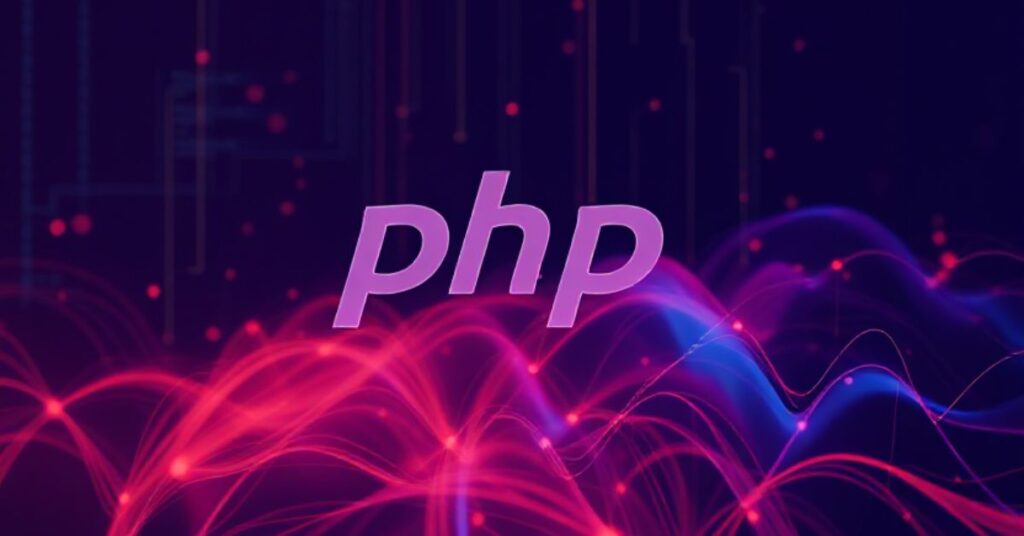
Yes, this error is common in specific PHP frameworks like ConcreteCMS, Magento, or others that rely on objects and collections for content management. These frameworks may return null when trying to load pages or blocks that don’t exist or are improperly configured.
It’s important to ensure that objects are correctly retrieved and checked before attempting to access their methods.
What Are the Consequences of Not Addressing This Error?
If you don’t address the “Call to a member function getCollectionParentId() on null” error, it can lead to the following issues:
- Application Crashes: The application may stop working entirely if the method is called on a null object.
- Unpredictable Behavior: Unhandled errors can lead to unexpected results, making the system unstable and harder to maintain.
- Poor User Experience: Visitors to your site or application may encounter blank pages or broken functionality, leading to frustration and potential loss of business.
Are There Any Best Practices to Avoid This Error?
To avoid the “call to a member function on null” error, follow these best practices:
- Consistent Object Initialization: Ensure that objects are always initialized before they are used. This reduces the chances of encountering null objects in your code.
- Comprehensive Error Handling: Always use try-catch blocks or other error-handling techniques to manage exceptions and provide users with meaningful error messages.
- Thorough Testing: Regularly test your code, especially database queries and object initialization, to identify potential issues before they affect your production environment.
Where Can I Find More Information About This Error?
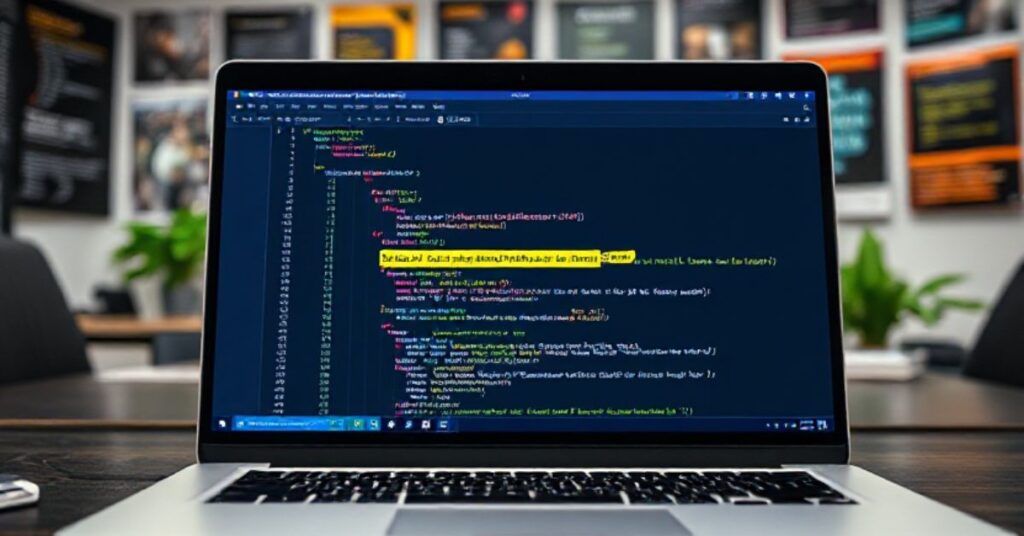
- Official Documentation: Review the documentation of the PHP framework or CMS you’re using. Most frameworks have detailed guides on handling objects and collections.
- Community Forums: Join developer communities like Stack Overflow or GitHub to ask questions and find solutions to common issues related to PHP errors.
- Online Articles: There are many blogs and articles that discuss common PHP errors and how to resolve them, such as the one you’re reading right now!
FAQs:
Can this error occur in other programming languages like JavaScript?
Yes, similar errors can occur in other languages if you try to invoke methods on undefined or null variables. Handling undefined variables is critical across all languages.
Is this error related to object-oriented programming in PHP?
Yes, it is specifically an object-oriented programming error in PHP, where a method is called on a variable that’s expected to be an object but is null. Proper object initialization is key to avoiding this.
How can I prevent this error when working with collections?
Always ensure that collections are properly loaded or initialized before performing operations like calling getCollectionParentId(). Verify that the collection is not empty or null.
What is the best practice for debugging this type of error?
Use debugging tools like Xdebug to inspect the value of variables and objects. Add logging to track the flow of data and detect where the null object is being introduced.
Why does this error often occur in CMS platforms like Magento or ConcreteCMS?
These platforms use object collections to manage data. If a collection fails to load or is empty, the variable becomes null, causing errors when methods are called on it.
How can I handle null values in PHP to avoid this error?
Implement null checks before accessing methods or properties. You can also use the isset() function or empty() to check if a variable is null or undefined.
What is the role of object initialization in avoiding this error?
Object initialization ensures that variables hold valid objects before methods are called. Without proper initialization, methods cannot be accessed, leading to the error.
Can database errors lead to a “call to a member function on null”?
Yes, if a database query fails or returns no results, the object being queried may be null, leading to this error when a method is called.
What is the main cause of the “Call to a member function on null” error in PHP?
The main cause is attempting to call a method on a variable or object that has not been properly initialized or is null. This often happens with uninstantiated objects or empty data returned from queries.
Conclusion
The “Call to a member function getCollectionParentId() on null” error can be frustrating, but it is usually easy to resolve with proper checks and error handling. By ensuring that your objects are correctly initialized and checking for null before invoking methods, you can prevent this error from disrupting your application. Happy coding!